Unleash Your Creativity: Mastering HTML, CSS, and JavaScript for Stunning Web Development ( explained )
"Discover the power of HTML, CSS, and JavaScript as you embark on a journey to create visually captivating and interactive web pages. This comprehensive online course will equip you with the essential skills and knowledge needed to craft stunning websites. From structuring content with HTML, designing beautiful layouts with CSS, to adding dynamic functionality with JavaScript, you'll gain the expertise to bring your web development visions to life. Join now and unlock the secrets to creating viral web experiences that captivate audiences worldwide.
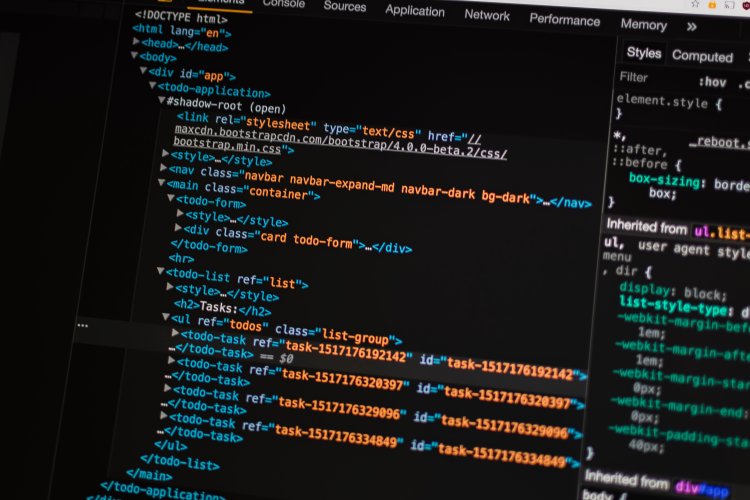
Lesson 1: Introduction to HTML
1.1. What is HTML? HTML (Hypertext Markup Language) is the standard markup language used to create the structure and content of web pages. It provides a set of tags that define the different elements of a web page, such as headings, paragraphs, images, links, and more.
1.2. Structure of an HTML Document An HTML document consists of several sections that define the structure and metadata of a web page. The main sections are:
<html>
<head>
<title>My Web Page</title>
</head>
<body>
<!-- Content goes here -->
</body>
</html>
The <!DOCTYPE>
declaration specifies the HTML version and document type. The <html>
element represents the root of an HTML page. The <head>
element contains metadata and other non-visible elements. The <body>
element contains the visible content of the web page.
1.3. Basic HTML Tags HTML tags are used to define the different elements and content within a web page. Some of the basic HTML tags include:
<h1>This is a Heading</h1>
<p>This is a paragraph.</p>
The <h1>
to <h6>
tags are used to define different levels of headings. The <p>
tag is used to define paragraphs of text.
1.4. Creating Hyperlinks Hyperlinks allow users to navigate between different web pages. In HTML, hyperlinks are created using the <a>
(anchor) tag. The most important attribute of the <a>
tag is href
, which specifies the target URL of the link. Example:
<a href="https://www.example.com">Click here</a>
This code creates a hyperlink with the text "Click here" that, when clicked, navigates to https://www.example.com
.
1.5. HTML Lists HTML provides two types of lists: ordered lists (<ol>
) and unordered lists (<ul>
). Both list types contain list items (<li>
) that define individual list entries. Ordered lists are numbered, while unordered lists are bulleted.
<ol>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ol>
<ul>
<li>Red</li>
<li>Green</li>
<li>Blue</li>
</ul>
The above code creates an ordered list with three items and an unordered list with three items.
1.6. HTML Images Images can be inserted into web pages using the <img>
tag. The src
attribute specifies the image file's URL, while the alt
attribute provides alternative text that is displayed if the image fails to load or for accessibility purposes.
<img src="image.jpg" alt="Description of the image">
This code inserts an image from the file "image.jpg" with the provided description as alternative text.
1.7. HTML Forms HTML forms are used to collect user input. Form elements such as text inputs, checkboxes, radio buttons, dropdown menus, and buttons are used to create interactive forms. When a user submits a form, the data can be processed using server-side or client-side scripting.
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<input type="submit" value="Submit">
</form>
The above code creates a simple form with input fields for name and email, and a submit button. The action
attribute specifies the URL where the form data should be submitted.
Lesson 2: CSS Fundamentals
2.1. Introduction to CSS CSS (Cascading Style Sheets) is a styling language used to describe the presentation of HTML documents. CSS allows you to control the appearance and layout of web pages, including elements such as fonts, colors, spacing, and positioning.
2.2. CSS Selectors CSS selectors are used to target specific HTML elements and apply styles to them. Common CSS selectors include:
- Element selectors: Select elements based on their tag names.
- Class selectors: Select elements with a specific class attribute.
- ID selectors: Select elements with a specific ID attribute.
- Attribute selectors: Select elements based on their attribute values.
/* Element selector */
p {
color: blue;
}
/* Class selector */
.my-class {
font-size: 20px;
}
/* ID selector */
#my-id {
background-color: yellow;
}
/* Attribute selector */
input[type="text"] {
border: 1px solid red;
}
In the above code, the styles are applied to different elements using element, class, ID, and attribute selectors.
2.3. CSS Box Model The CSS box model describes how elements are rendered on a web page. It consists of four layers: content, padding, border, and margin. Understanding the box model is crucial for controlling the size and spacing of elements.
.box {
width: 200px;
height: 100px;
padding: 20px;
border: 1px solid black;
margin: 10px;
}
In the code above, a box with a width of 200 pixels and a height of 100 pixels is created. Padding adds space inside the box, border creates a visible boundary, and margin adds space around the box.
2.4. CSS Colors and Backgrounds CSS provides various ways to specify colors, including keywords, hexadecimal values, RGB, and HSL values. Background colors and images can be applied to elements to enhance their visual appearance.
.heading {
color: #ff0000;
background-color: yellow;
}
.link {
color: rgb(0, 128, 0);
background-image: url("background.jpg");
}
In the above code, different color formats and background images are applied to headings and links.
2.5. CSS Text Properties CSS offers numerous properties for controlling the styling of text, such as font family, size, weight, style, color, alignment, and spacing. These properties allow you to customize the typography of your web page.
.text {
font-family: Arial, sans-serif;
font-size: 16px;
font-weight: bold;
color: #333333;
text-align: center;
line-height: 1.5;
letter-spacing: 1px;
}
The above code demonstrates various text properties that can be applied to an element with the class "text".
2.6. CSS Layouts and Positioning CSS provides different techniques for creating layouts and positioning elements on a web page. This includes using static positioning, relative positioning, absolute positioning, and fixed positioning. Floats and CSS flexbox and grid layouts are also covered.
.container {
width: 800px;
margin: 0 auto;
}
.box {
position: relative;
top: 50px;
left: 20px;
float: left;
}
.flex-container {
display: flex;
justify-content: center;
align-items: center;
}
.grid-container {
display: grid;
grid-template-columns: 1fr 1fr;
grid-gap: 10px;
}
In the above code, different layout and positioning techniques, such as floating, flexbox, and grid, are demonstrated.
2.7. CSS Transitions and Animations CSS transitions and animations allow you to add motion and interactivity to your web page. Transitions create smooth effects when properties change, while animations define keyframes and animations that can be applied to elements.
.button {
background-color: blue;
color: white;
transition: background-color 0.3s ease;
}
.button:hover {
background-color: red;
}
@keyframes slide-in {
from {
transform: translateX(-100%);
}
to {
transform: translateX(0);
}
}
.slide {
animation: slide-in 1s ease-in-out;
}
In the above code, a button with a transition effect and an element with a sliding animation are demonstrated.
Lesson 3: JavaScript Fundamentals
3.1. Introduction to JavaScript JavaScript is a programming language that adds interactivity and dynamic behavior to web pages. It is primarily used for client-side scripting, enabling actions to be performed directly in the user's browser.
3.2. Variables and Data Types Variables are used to store and manipulate data in JavaScript. JavaScript has several built-in data types, including:
string
: Represents text data.number
: Represents numeric values.boolean
: Represents true or false values.array
: Represents an ordered collection of values.object
: Represents a collection of key-value pairs.
// Variable declaration and assignment
let name = "John";
let age = 25;
let isStudent = true;
let fruits = ["apple", "banana", "orange"];
let person = { name: "John", age: 25 };
In the above code, variables are declared using the let
keyword and assigned values of different data types.
3.3. Operators and Expressions JavaScript provides various operators for performing operations on values, such as arithmetic operators (+, -, *, /), comparison operators (>, <, ===), logical operators (&&, ||), and more. Expressions combine variables, values, and operators to produce a result.
let num1 = 10;
let num2 = 5;
let sum = num1 + num2;
let isGreater = num1 > num2;
let result = (num1 * 2) + (num2 * 3);
The above code demonstrates the use of arithmetic, comparison, and assignment operators to perform calculations and evaluate conditions.
3.4. Control Flow and Conditional Statements Conditional statements allow you to make decisions and execute different blocks of code based on specified conditions. JavaScript provides if
, else if
, and else
statements for branching logic.
let age = 18;
if (age < 13) {
console.log("Child");
} else if (age >= 13 && age < 18) {
console.log("Teenager");
} else {
console.log("Adult");
}
In the above code, different conditions are checked using the if
, else if
, and else
statements to determine the appropriate message to display.
3.5. Loops Loops allow you to repeat a block of code multiple times. JavaScript provides several loop structures, including for
loops and while
loops.
// For loop
for (let i = 1; i <= 5; i++) {
console.log(i);
}
// While loop
let count = 0;
while (count < 5) {
console.log(count);
count++;
}
The above code demonstrates the use of a for
loop and a while
loop to iterate over a set of values.
3.6. Functions Functions allow you to group code into reusable blocks and execute them when needed. JavaScript functions can accept parameters and return values.
// Function declaration
function greet(name) {
return "Hello, " + name + "!";
}
// Function call
let message = greet("John");
console.log(message);
In the above code, a function named greet
is declared and then called with an argument to produce a greeting message.
3.7. DOM Manipulation The Document Object Model (DOM) represents the structure of an HTML document. JavaScript can interact with the DOM to manipulate elements and respond to user actions.
// Change text content
let heading = document.getElementById("myHeading");
heading.textContent = "New Heading";
// Add event listener
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button clicked!");
});
The above code demonstrates changing the text content of an element and adding an event listener to a button.
What's Your Reaction?
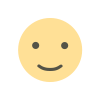

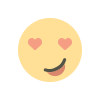
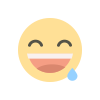
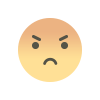
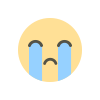
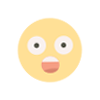