Squaring a Value
Could the C language use a square() function or operator? Continue reading →
The C language lacks an operator that squares a value or, more generally, raises a value to a specific power. To do so, use the pow() function. Yet I’m finding the need for a square() function as I explore some interesting and obscure mathematical thingies.
In my digital travels, I’ve seen a few “square” or “power” operators. Perhaps the most common is the caret, ^
, as in 2^5
which raises two to the fifth power. Remember that in C, the ^
is the bitwise exclusive OR operator.
Another exponent operator I’ve seen is **
as in 2**5
, which raises two to the fifth. In C, **
is used to identify a pointer to a pointer (an array of pointers).
The lack of an exponent operator in C means that programmers use the pow() function to raise a value to a specific power:
pow(2,5);
The above statement raises two to the fifth power. It requires that you include the math.h
header file. On some systems, you must also remember to link in the math library (-lm
) on the command line.
Squaring a value happens frequently in math. The way I often code it in C is to write alpha*alpha
as opposed to pow(alpha,2);
I would guess that alpha*alpha
probably calculates faster internally.
The following code demonstrates both methods to square a value:
2024_03_16-Lesson-a.c
#include#include int main() { int alpha = 5; printf("Alpha = %d\n",alpha); printf("Alpha squared is %d\n",alpha*alpha); printf("Alpha squared is %.f\n",pow(alpha,2) ); return 0; }
Here is the output:
Alpha = 5
Alpha squared is 25
Alpha squared is 25
If I’m desperate for a square() function, I could write one. But instead, I think it would be better and more nerdy to code a macro, as shown in this update to the code:
2024_03_16-Lesson-b.c
#include#include #define squared(a) a*a int main() { int alpha = 5; printf("Alpha = %d\n",alpha); printf("Alpha squared is %d\n",alpha*alpha); printf("Alpha squared is %.f\n",pow(alpha,2) ); printf("Alpha squared is %d\n",squared(alpha) ); return 0; }
The squared() “function” is really a macro. It accepts an argument, represented as a
in the definition, then replaces it with a*a
:
#define squared(a) a*a
In the code, the final printf() statement is translated by the precompiler to this:
printf("Alpha squared is %d\n",squared(alpha*alpha) );
The output is the same as the first printf() statement. I don’t think the macro saves any time, but it does add to readability, which is what I wanted.
Well, what I’d really want is an exponent operator, but that’s never going to happen in the C language.
What's Your Reaction?
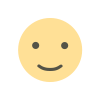

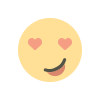
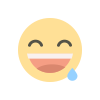
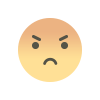
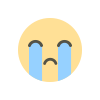
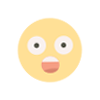