Practical JavaScript: A Hands-On Guide to Web Development
Discover essential JavaScript concepts, practical projects, best practices, and resources in this comprehensive guide. From fundamental syntax and data manipulation to advanced topics like DOM manipulation, asynchronous programming, and performance optimization, this book covers everything you need to become a proficient JavaScript developer.
With this comprehensive guide that covers everything from fundamental syntax to advanced techniques and best practices. Whether you're a beginner or an experienced developer, you'll find valuable insights, practical projects, and recommended resources to hone your skills and build real-world applications. Explore essential JavaScript concepts, tackle practical projects like building a To-Do List application and a weather forecast application with API integration, and learn about debugging techniques, performance optimization, and more. With clear explanations, code examples, and recommended resources, this book is your ultimate companion on your journey to mastering JavaScript development.
Chapter 1: Introduction to JavaScript
- What is JavaScript?
- Why JavaScript is important in web development?
- Basic syntax and structure of JavaScript code.
Chapter 2: Variables, Data Types, and Operators
- Declaring and initializing variables.
- Data types: strings, numbers, booleans, arrays, and objects.
- Arithmetic, assignment, comparison, and logical operators.
Chapter 3: Control Flow and Functions
- Conditional statements: if-else, switch-case.
- Loops: for, while, do-while.
- Functions: defining functions, parameters, return values, and function expressions.
Chapter 4: Arrays and Objects
- Working with arrays: creating, accessing, modifying, and iterating.
- Introduction to objects: properties and methods.
- Manipulating objects: adding, deleting, and updating properties.
Chapter 5: DOM Manipulation
- Understanding the Document Object Model (DOM).
- Accessing and manipulating DOM elements.
- Handling events: click, submit, hover, etc.
Chapter 6: Asynchronous JavaScript
- Introduction to asynchronous programming.
- Working with callbacks, promises, and async/await.
- Making asynchronous requests using Fetch API.
Chapter 7: Practical Projects
- Building a To-Do List application.
- Creating a simple game using JavaScript.
- Developing a weather forecast application with API integration.
Chapter 8: Best Practices and Tips
- Writing clean and maintainable code.
- Debugging JavaScript code effectively.
- Performance optimization techniques.
Chapter 9: Resources and Further Learning
- Recommended books, websites, and online courses for mastering JavaScript.
- Useful tools and libraries for JavaScript development.
Chapter 1: Introduction to JavaScript
JavaScript is a high-level programming language commonly used for web development. It was initially created by Brendan Eich at Netscape in 1995 and has since become one of the most widely used programming languages in the world.
JavaScript plays a crucial role in web development for several reasons:
-
Client-Side Interactivity: JavaScript allows developers to create dynamic and interactive elements on web pages. With JavaScript, you can manipulate HTML and CSS, handle user interactions, and update content in real-time without needing to reload the entire page.
-
Cross-Platform Compatibility: JavaScript is supported by all major web browsers, including Chrome, Firefox, Safari, and Edge, making it a versatile choice for building web applications that work across different platforms and devices.
-
Rich Ecosystem: JavaScript has a vast ecosystem of libraries, frameworks, and tools that simplify and enhance web development. Popular libraries like jQuery, React, and Vue.js, as well as frameworks like Angular and Node.js, offer powerful features for building complex web applications.
-
Server-Side Development: With the introduction of Node.js, JavaScript can now be used for server-side development as well. This allows developers to use a single language (JavaScript) for both client-side and server-side development, resulting in a more streamlined development process.
JavaScript has a simple and straightforward syntax, making it accessible to beginners while still offering powerful features for experienced developers. Here's a brief overview of the basic syntax and structure of JavaScript code:
-
Variables: Variables are used to store data values. They are declared using the
var
,let
, orconst
keywords. -
Data Types: JavaScript supports various data types, including strings, numbers, booleans, arrays, objects, and more.
-
Operators: JavaScript includes arithmetic, assignment, comparison, and logical operators for performing operations on variables and values.
-
Functions: Functions are reusable blocks of code that perform a specific task. They are defined using the
function
keyword and can accept parameters and return values. -
Control Flow: JavaScript includes conditional statements (such as
if
,else
, andswitch
) and loops (such asfor
,while
, anddo-while
) for controlling the flow of execution in a program.
Chapter 2: Variables, Data Types, and Operators
In this chapter, we'll dive deeper into the world of JavaScript variables, data types, and operators.
Declaring and Initializing Variables
Variables are containers for storing data values. In JavaScript, you can declare variables using the var
, let
, or const
keywords. Here's how you can declare and initialize variables:
- var: Variables declared with
var
are function-scoped or globally scoped. They can be reassigned and redeclared within their scope. - let: Variables declared with
let
are block-scoped. They can be reassigned but not redeclared within the same scope. - const: Variables declared with
const
are also block-scoped but cannot be reassigned once initialized. However, they can still be mutated if they hold a reference to an object or array.
var age = 25;
let name = "John";
const PI = 3.14;
Data Types: Strings, Numbers, Booleans, Arrays, and Objects
JavaScript supports various data types for representing different kinds of values:
- Strings: Textual data enclosed within single or double quotes.
- Numbers: Numeric data, including integers and floating-point numbers.
- Booleans: Logical values representing true or false.
- Arrays: Ordered collections of values, indexed by numerical positions.
- Objects: Unordered collections of key-value pairs, where keys are strings and values can be of any data type.
let message = "Hello, world!";
let age = 25;
let isMale = true;
let colors = ["red", "green", "blue"];
let person = { name: "John", age: 30 };
Arithmetic, Assignment, Comparison, and Logical Operators
JavaScript provides various operators for performing operations on variables and values:
- Arithmetic Operators: Used for performing arithmetic operations such as addition, subtraction, multiplication, division, and modulus.
- Assignment Operators: Used for assigning values to variables.
- Comparison Operators: Used for comparing values and returning true or false.
- Logical Operators: Used for combining or negating boolean values.
let x = 10;
let y = 5;
// Arithmetic operators
let sum = x + y;
let difference = x - y;
let product = x * y;
let quotient = x / y;
let remainder = x % y;
// Assignment operators
let z = 10;
z += 5; // equivalent to z = z + 5
// Comparison operators
console.log(x > y); // true
console.log(x === y); // false
// Logical operators
let isTrue = true;
let isFalse = false;
console.log(isTrue && isFalse); // false
console.log(isTrue || isFalse); // true
console.log(!isTrue); // false
Chapter 3: Control Flow and Functions
In this chapter, we'll explore control flow statements and functions, which are essential concepts in JavaScript for controlling the flow of execution and organizing our code.
Conditional Statements: if-else, switch-case
Conditional statements allow us to execute different blocks of code based on specified conditions:
- if-else: Executes a block of code if a specified condition is true, and another block of code if the condition is false.
let x = 10;
if (x > 0) {
console.log("x is positive");
} else {
console.log("x is non-positive");
}
- switch-case: Evaluates an expression and executes the corresponding case statement based on its value.
let day = "Monday";
switch (day) {
case "Monday":
console.log("It's Monday!");
break;
case "Tuesday":
console.log("It's Tuesday!");
break;
default:
console.log("It's not Monday or Tuesday.");
}
Loops: for, while, do-while
Loops allow us to execute a block of code repeatedly:
- for: Executes a block of code a specified number of times.
for (let i = 0; i < 5; i++) {
console.log(i);
}
- while: Executes a block of code while a specified condition is true.
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
- do-while: Similar to the while loop, but the block of code is executed at least once before the condition is tested.
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
Functions: Defining Functions, Parameters, Return Values, and Function Expressions
Functions are blocks of code that perform a specific task. They help organize code and make it reusable:
- Defining Functions: Functions are defined using the
function
keyword, followed by the function name and parameters (if any), and the function body enclosed in curly braces.
function greet(name) {
console.log("Hello, " + name + "!");
}
- Parameters: Functions can accept parameters, which are variables passed to the function when it is called.
function add(x, y) {
return x + y;
}
- Return Values: Functions can return a value using the
return
keyword. This value can be used in other parts of the code.
function multiply(x, y) {
return x * y;
}
let result = multiply(2, 3); // result is 6
- Function Expressions: Functions can also be defined using function expressions, where a function is assigned to a variable.
let greet = function(name) {
console.log("Hello, " + name + "!");
};
Understanding control flow statements and functions is essential for writing structured and organized JavaScript code.
Chapter 4: Arrays and Objects
In this chapter, we'll explore two important data structures in JavaScript: arrays and objects.
Working with Arrays: Creating, Accessing, Modifying, and Iterating
Arrays are ordered collections of values, indexed by numerical positions. We'll cover various operations you can perform with arrays:
- Creating Arrays: Arrays can be created using square brackets
[]
and can contain any combination of values, including numbers, strings, booleans, or even other arrays.
let numbers = [1, 2, 3, 4, 5];
let colors = ["red", "green", "blue"];
- Accessing Array Elements: Array elements can be accessed using their index, starting from 0 for the first element.
console.log(numbers[0]); // Output: 1
- Modifying Arrays: Arrays are mutable, meaning you can change their elements by assigning new values or using methods like
push
,pop
,shift
, andunshift
.
colors[0] = "yellow"; // Modify the first element
colors.push("orange"); // Add a new element to the end
- Iterating Over Arrays: You can loop through arrays using various methods like
for
loops,forEach
,map
,filter
, andreduce
.
for (let i = 0; i < colors.length; i++) {
console.log(colors[i]);
}
Introduction to Objects: Properties and Methods
Objects are unordered collections of key-value pairs, where keys are strings and values can be of any data type. Here's how you can work with objects:
- Creating Objects: Objects can be created using curly braces
{}
and consist of key-value pairs separated by colons:
.
let person = {
name: "John",
age: 30,
isMale: true
};
- Accessing Object Properties: Object properties can be accessed using dot notation or bracket notation.
console.log(person.name); // Dot notation
console.log(person["age"]); // Bracket notation
- Manipulating Objects: Objects are mutable, allowing you to add, delete, or update properties dynamically.
person.job = "Developer"; // Add a new property
delete person.isMale; // Delete a property
person.age = 31; // Update an existing property
Understanding arrays and objects is essential for handling and manipulating data in JavaScript.
Chapter 5: DOM Manipulation
In this chapter, we'll dive into the Document Object Model (DOM) and learn how to manipulate it using JavaScript.
Understanding the Document Object Model (DOM)
The Document Object Model (DOM) is a programming interface for web documents. It represents the structure of an HTML document as a tree of nodes, allowing JavaScript to interact with and manipulate the content, structure, and style of a web page.
Accessing and Manipulating DOM Elements
JavaScript provides various methods for accessing and manipulating DOM elements:
- getElementById: Retrieves an element by its unique ID attribute.
- getElementsByClassName: Retrieves elements by their class name.
- getElementsByTagName: Retrieves elements by their tag name.
- querySelector: Retrieves the first element that matches a specific CSS selector.
- querySelectorAll: Retrieves all elements that match a specific CSS selector.
// Example: Accessing DOM elements
let elementById = document.getElementById("myElement");
let elementsByClassName = document.getElementsByClassName("myClass");
let elementsByTagName = document.getElementsByTagName("div");
let firstElementBySelector = document.querySelector(".myClass");
let allElementsBySelector = document.querySelectorAll(".myClass");
Once we've accessed DOM elements, we can manipulate them by changing their properties, attributes, or content using JavaScript.
Handling Events: click, submit, hover, etc.
Events are actions or occurrences that happen in the browser, such as mouse clicks, keyboard inputs, or page loading. JavaScript allows us to handle these events and execute code in response to them.
// Example: Handling click event
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
Commonly used events include click, submit, change, mouseover, mouseout, keydown, keyup, and more. By attaching event listeners to DOM elements, we can create interactive and responsive web applications.
Understanding DOM manipulation is crucial for dynamic web development.
Chapter 6: Asynchronous JavaScript
In this chapter, we'll explore asynchronous programming in JavaScript, which allows us to execute code concurrently and handle operations that may take some time to complete without blocking the main execution thread.
Introduction to Asynchronous Programming
Asynchronous programming in JavaScript enables non-blocking behavior, meaning that code can continue to execute while waiting for asynchronous operations to complete. This is essential for tasks like fetching data from an external server, reading files, or handling user interactions without freezing the user interface.
Working with Callbacks, Promises, and async/await
JavaScript provides several mechanisms for handling asynchronous operations:
- Callbacks: Callback functions are functions that are passed as arguments to other functions and are executed once the asynchronous operation completes.
function fetchData(callback) {
setTimeout(() => {
callback("Data received!");
}, 1000);
}
fetchData((data) => {
console.log(data);
});
- Promises: Promises are objects representing the eventual completion or failure of an asynchronous operation. They allow us to chain multiple asynchronous operations and handle success and error cases more easily.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data received!");
}, 1000);
});
}
fetchData()
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
- async/await: async functions allow us to write asynchronous code in a synchronous manner using the
await
keyword. This makes asynchronous code easier to read and understand.
async function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data received!");
}, 1000);
});
}
async function getData() {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(error);
}
}
getData();
Making Asynchronous Requests using Fetch API
The Fetch API provides an interface for fetching resources asynchronously across the network. It provides a more powerful and flexible way to make HTTP requests compared to traditional approaches like XMLHttpRequest.
fetch("https://api.example.com/data")
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Understanding asynchronous programming is crucial for building modern web applications that rely on fetching data from servers or performing other time-consuming tasks without blocking the main thread.
Chapter 7: Practical Projects
In this chapter, we'll embark on three practical projects using JavaScript to apply the concepts we've learned and build real-world applications.
1. Building a To-Do List Application
A To-Do List application allows users to create, manage, and organize tasks they need to accomplish. We'll implement features like adding tasks, marking tasks as completed, deleting tasks, and filtering tasks by status.
Tutorial Highlights:
- Set up the project structure and HTML layout.
- Use JavaScript to add interactivity and functionality to the To-Do List.
- Implement event listeners for adding, completing, and deleting tasks.
- Store tasks in the browser's localStorage for persistence.
- Style the application using CSS for a better user experience.
Expected Results:
- Users can add new tasks to the list.
- Users can mark tasks as completed or delete them.
- Tasks are stored locally and persist even after page refresh.
Download Source Code of To-Do List Application Javascript
2. Creating a Simple Game using JavaScript
We'll create a simple game using JavaScript, such as a guessing game, a memory matching game, or a rock-paper-scissors game. This project will focus on implementing game logic, handling user input, and updating the game state dynamically.
Tutorial Highlights:
- Design the game layout using HTML and CSS.
- Implement game logic and functionality using JavaScript.
- Handle user interactions and input events.
- Update the game interface dynamically based on the game state.
- Add scoring, timers, or other features to enhance gameplay.
Expected Results:
- Users can interact with the game and play it successfully.
- The game responds to user input and updates the interface accordingly.
- Users receive feedback or notifications about their game progress.
Download Source Code of Simple Game using JavaScript
3. Developing a Weather Forecast Application with API Integration
We'll create a weather forecast application that retrieves weather data from a public API and displays it to the user. This project will involve making asynchronous requests to the API, parsing the JSON response, and presenting the weather information in a user-friendly format.
Tutorial Highlights:
- Set up the project structure and HTML layout.
- Use JavaScript to make asynchronous requests to a weather API.
- Parse the JSON response and extract relevant weather data.
- Display the weather forecast information on the web page.
- Enhance the application with additional features like location-based weather or multiple-day forecasts.
Expected Results:
- Users can enter a location and view the current weather forecast.
- The application retrieves and displays weather data from the API accurately.
- Users can see additional details like temperature, humidity, and wind speed.
Download Source Code of Weather Forecast Application with API Integration using JavaScript
By completing these practical projects, you'll gain hands-on experience in applying JavaScript concepts to real-world scenarios, honing your skills, and building valuable portfolio projects.
Chapter 8: Best Practices and Tips
In this chapter, we'll cover best practices and tips for writing clean, maintainable, and efficient JavaScript code.
Writing Clean and Maintainable Code:
- Use meaningful variable names and comments to enhance code readability.
- Follow consistent coding conventions and indentation styles.
- Break down complex tasks into smaller, reusable functions for better organization.
- Avoid nested callbacks or deeply nested structures to improve code maintainability.
Debugging JavaScript Code Effectively:
- Utilize browser developer tools like Chrome DevTools or Firefox Developer Tools for debugging.
- Use console.log() statements strategically to log variable values and trace code execution.
- Employ breakpoints to pause code execution and inspect variables at specific points.
- Leverage browser extensions like JavaScript linters or code validators to catch syntax errors and potential bugs early.
Performance Optimization Techniques:
- Minimize DOM manipulation and avoid unnecessary reflows or repaints.
- Optimize loops and algorithms to reduce time complexity and improve performance.
- Use event delegation to minimize the number of event listeners, especially for large DOM structures.
- Implement lazy loading for resources like images or scripts to reduce initial page load times.
Chapter 9: Resources and Further Learning
In this chapter, we'll explore recommended books, websites, online courses, tools, and libraries for mastering JavaScript and advancing your skills.
Recommended Books:
- "Eloquent JavaScript" by Marijn Haverbeke
- "JavaScript: The Good Parts" by Douglas Crockford
- "You Don't Know JS" series by Kyle Simpson
- "JavaScript: The Definitive Guide" by David Flanagan
Websites and Online Courses:
- MDN Web Docs: Comprehensive documentation and tutorials for JavaScript.
- freeCodeCamp: Interactive coding challenges and projects for learning JavaScript.
- Codecademy: Online courses covering various aspects of JavaScript development.
- Udemy and Coursera: Platforms offering JavaScript courses taught by industry experts.
Useful Tools and Libraries:
- ESLint: JavaScript linter for identifying and fixing code errors and enforcing coding standards.
- Babel: JavaScript compiler for converting modern ECMAScript code to browser-compatible versions.
- Lodash: Utility library providing helpful functions for simplifying JavaScript code.
- Axios: Promise-based HTTP client for making AJAX requests and handling responses easily.
By incorporating these best practices, tips, and resources into your JavaScript development workflow, you'll enhance your coding skills, write more efficient code, and continue learning and growing as a developer.
Files to Download:
Files
What's Your Reaction?
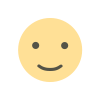

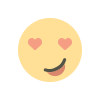
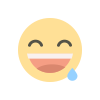
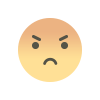
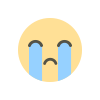
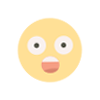