Positive Negative Positive Negative – Solution
This month’s Exercise presents what I often refer to as a code “toggle.” Many paths lead to a solution. Because of my interest in coding various math equations, my goal is to craft a solution distilled into a single expression. … Continue reading →
This month’s Exercise presents what I often refer to as a code “toggle.” Many paths lead to a solution.
Because of my interest in coding various math equations, my goal is to craft a solution distilled into a single expression. Turns out that all of my solutions easily fit into a single expression, even the one that didn’t work.
For my first solution, I use the modulus operator in a ternary expression:
2024_02-Exercise-a.c
#includeint main() { int a; for( a=0; a<14; a++ ) printf("%d\n",a%2?-1:1); return(0); }
Variable a
loops from 0 through 14. The expression in the printf() statement tests for odd and even values of a
. For odd values, -1 is output; for even values 1 is output.
For my second solution, I went binary. Bits toggle between two values, which can also be set in a single expression:
2024_02-Exercise-b.c
#includeint main() { int a; for( a=0; a<14; a++ ) printf("%d\n",a&0x01?-1:1); return(0); }
The bitwise manipulation a&0x01
masks bits 7 through 2 in 16-bit integer value. What remains is the right-most bit, which alternates between zero and one for increasing values of variable a
. The effect is the same as my first solution, resulting in TRUE/FALSE condition in a ternary operation.
For my last solution, I turned to the C23 standard and its variable integer width data specification, _BitInt(n). I figured that I’d be truly clever and create a one-bit integer.
2024_02-Exercise-c.c (C23)
#includeint main() { int a; _BitInt(2) toggle; toggle = 0; for( a=0; a<14; a++ ) { printf("%d\n",(int)toggle); toggle++; } return(0); }
As you can see in the source code, the _BitInt(n) declaration uses two bits, not one. Apparently the C23 standard doesn’t allow for single bit integers, at least on the clang-15 compiler I’m using.
Here is the output from my final attempt:
0
1
-2
-1
0
1
-2
-1
0
1
-2
-1
0
1
The values oscillate, as any integer would between its limits. The range for a 2-bit integer in C23 is from -2 through 1. Yes, it’s a signed value. I tried casting the variable to unsigned, but it didn’t work. So much for being clever. (And remember that you must use a compatible C23 compiler with the -std=c2x
switch.)
I hope you came up with something interesting or different. This type of exercise is one that yields a variety of results — perhaps even including a few obfuscated examples. This potential is why I so enjoy the C language.
What's Your Reaction?
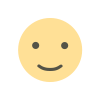

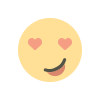
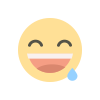
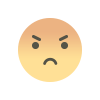
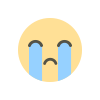
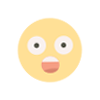